Assertion Test Components
Assertions are a type of component that you can add to a test using the Composer. To learn how to access the components and create a test using the Composer see Writing API Tests with the Composer.
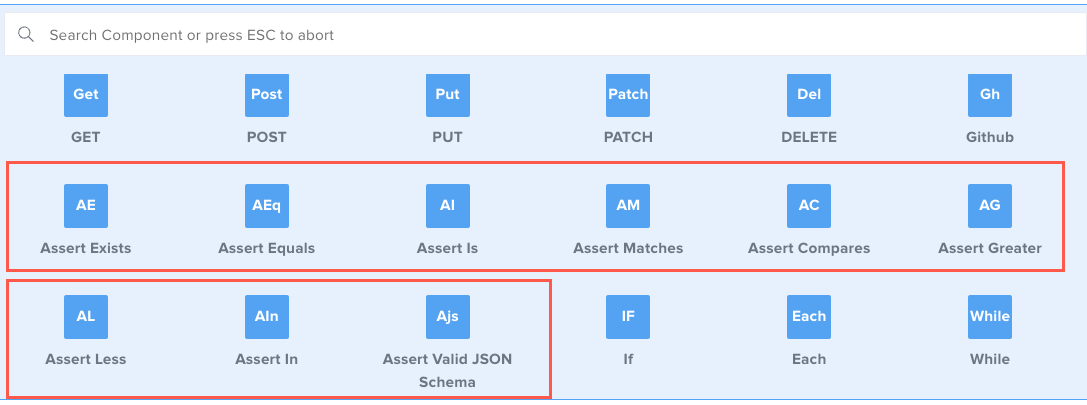
What You'll Need
- A Sauce Labs account (Log in or sign up for a free trial license).
- Familiarity with the API Testing Composer.
Assert Compares
Allows you to compare two payloads in terms of text, structure or values.
Parameters
Fields | |
Expression 1 | | REQUIRED | STRING | The first payload you want to compare. |
Expression 2 | | REQUIRED | STRING | The second payload you want to compare. |
Mode | | REQUIRED | Text, values, structure | The comparator you wish to use. |
Strict | | OPTIONAL | Yes, No | Comparison includes data types. |
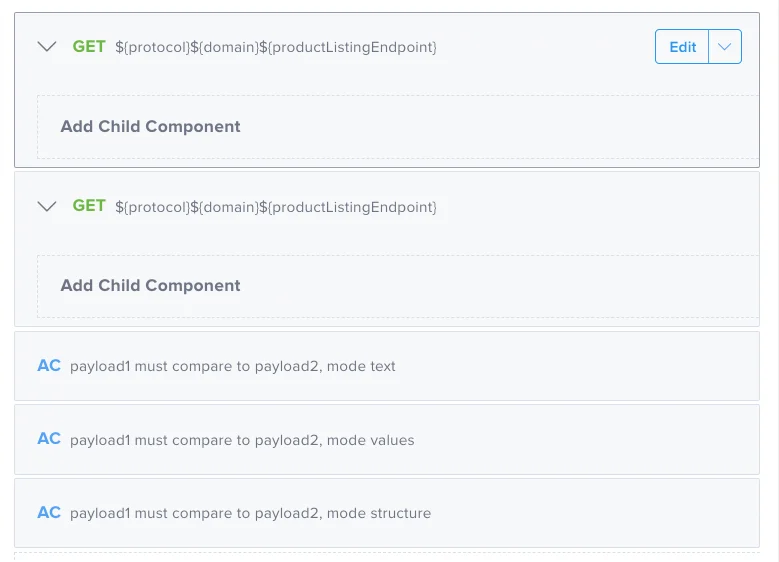
Code View Examples
- id: assert-compares
expression1: payload1
expression2: payload2
mode: text
strict: 'false'
- id: assert-compares
expression1: payload1
expression2: payload2
mode: values
strict: 'false'
- id: assert-compares
expression1: payload1
expression2: payload2
mode: structure
strict: 'false'
Assert Contains
This assertion is used to check if the element described by the expression contains a specific substring. For example, to test the word Uber is in Uber's product names (UberX, UberBlack, UberPool).
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Value | | REQUIRED | String, Number, Boolean | The value we want to compare the expression to. |
Type | | OPTIONAL | Auto, String, Number, Boolean | The type of the value. |
Code View Examples
- id: assert-contains
expression: data.url
value: domain.com
- id: assert-contains
expression: data.id
value: ${id}
Assert Equals
This assertion is used to check if the element value described by the expression is equal to a specific value. A direct one-to-one comparison.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Value | | REQUIRED | String, Number, Boolean | The value we want to compare the expression to. |
Type | | OPTIONAL | Auto, String, Number, Boolean | The type of the value. |
Code View Examples
- id: assert-equals
expression: data.code
value: '500'
- id: assert-equals
expression: data.code
value: 500
Assert Exists
This assertion is used to check if the element described by the expression exists. The presence of the element, even empty, is enough to consider it a valid assertion.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Code View Examples
- id: assert-exists
expression: data.id
Assert Greater
This assertion is used to check if the element value described by the expression is greater than a proposed value. The values can be compared as a string or number.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Value | | REQUIRED | String, Number | The value we want to compare the expression to. |
Code View Examples
- id: assert-greater
expression: data.code
value: 4503
Assert In
This assertion is used to check if the element described by the expression matches at least one item from a given list. For example, the category of a product is one of the approved categories such as men, women, or children.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Value | | REQUIRED | String, Number | The value we want to compare the expression to. |
Code View Examples
- id: assert-in
expression: data.type
value:
- ebook
- paperbook
- id: assert-in
expression: data.price
value:
- '5.50'
- '7'
- '9.79'
Assert Is
This assertion is used to check if the value of the element defined by the expression belongs to a specific type. This is one of the more commonly used assertions because it can be used to verify various things such as whole numbers, email addresses, phone numbers, URLs, and so forth.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Type | | REQUIRED | Integer, float, url, boolean, phone, email, map, array | The data type of the value. |
Code View Examples
- id: assert-is
expression: data.id
type: integer
Assert Less
This assertion is used to check if the element value described by the expression is less than a proposed value. The values can be compared as a string or number.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Value | | REQUIRED | String, Number | The value we want to compare the expression to. |
Code View Examples
- id: assert-less
expression: data.code
value: 4503
Assert Matches
This assertion is used to check if the element value described by the expression matches a knowledge base of some kind (e.g, U.S. state or zip code). This also gives you the ability to write your own regex (regular expression).
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
Type | | REQUIRED | 'regex', 'US Zipcode', 'USState', 'credit card', 'country codes', 'currency codes' | The data type of the value. |
Regex value | | REQUIRED, if type is 'regex' | String | Specify the regular expression you want to use for checking the expression. |
Code View Examples
- id: assert-matches
expression: data.zipcode
type: us_zipcodes
Assert Valid JSON Schema
This assertion is used to validate a JSON schema, based on the provided schema definition.
Parameters
Fields | |
Expression | | REQUIRED | Expression | The path to the element we want to operate on (e.g., |
JSON Schema | | REQUIRED | JSON schema definition | The JSON schema definition. This will be used to validate the JSON passed in the expression field. |
Code View Examples
- id: set
var: json_success
mode: lang
lang: template
body: '{ "rectangle" : { "a" : 15, "b" : 5 } }'
- id: assert-valid-jsonschema
expression: json_success
body: |-
{
"type": "object",
"properties": {
"rectangle": {
"$ref": "#/definitions/Rectangle"
}
},
"definitions": {
"size": {
"type": "number",
"minimum": 0
},
"Rectangle": {
"type": "object",
"properties": {
"a": {
"$ref": "#/definitions/size"
},
"b": {
"$ref": "#/definitions/size"
}
}
}
}
}
Assertion Common Fields
Comment
| OPTIONAL | String |
Add comment messages in the form of a string data type.Modifier
| OPTIONAL | 'not' |
The assertion is considered verified if it does not pass.Not available in Assert Compares and Assert Valid JSON Schema
Execute if item exists
| OPTIONAL | yes, no |
The assertion is evaluated only if the element exists. This is useful when the element does not always exist.Not available in Assert Compares, Assert Exists and Assert Valid JSON Schema.
Level
| OPTIONAL | error, warning |
Specify if the assertion fails whether it should be considered anerror
or just a warning
.
A warning will not trigger alerts, such as email.
Stop test if fails
| OPTIONAL | Yes, No |
The test will be immediately stopped if the assertion fails.Not available in Assert Valid JSON Schema.