Logical Test Components
Logical components are a type of component that you can add to a test using the Compose tab (aka Composer). To learn how to access the components and create a test using the Composer see Writing API Tests with the Composer.
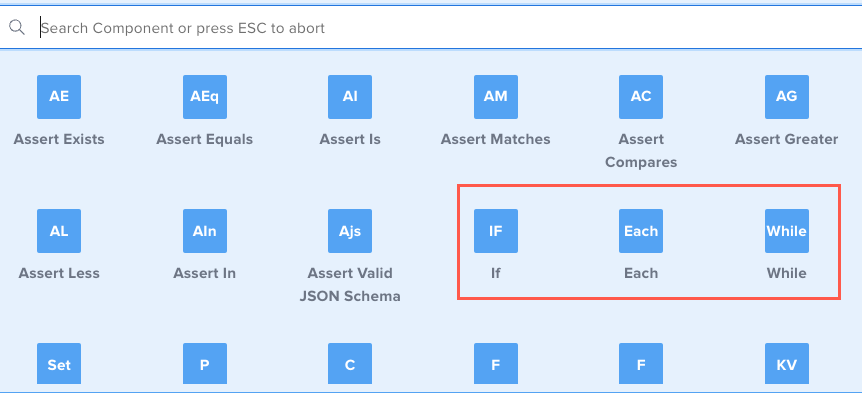
What You'll Need
- A Sauce Labs account (Log in or sign up for a free trial license).
- An existing API Testing Project. For details on how to create one, see API Testing Quickstart.
- Familiarity with the API Testing Composer.
Logical Components
Each
Allows you to iterate over a collection of elements and execute the piece of code for each element.
Parameters
Fields | |
Expression | | REQUIRED | EXPRESSION | The path of the collection you want to iterate on. |
Examples

vector
item is an integer value.Legs Collection Example
{
"legs": [
{
"vector": 1
},
{
"vector": 3
}
]
}
_1
, _2
, and so on.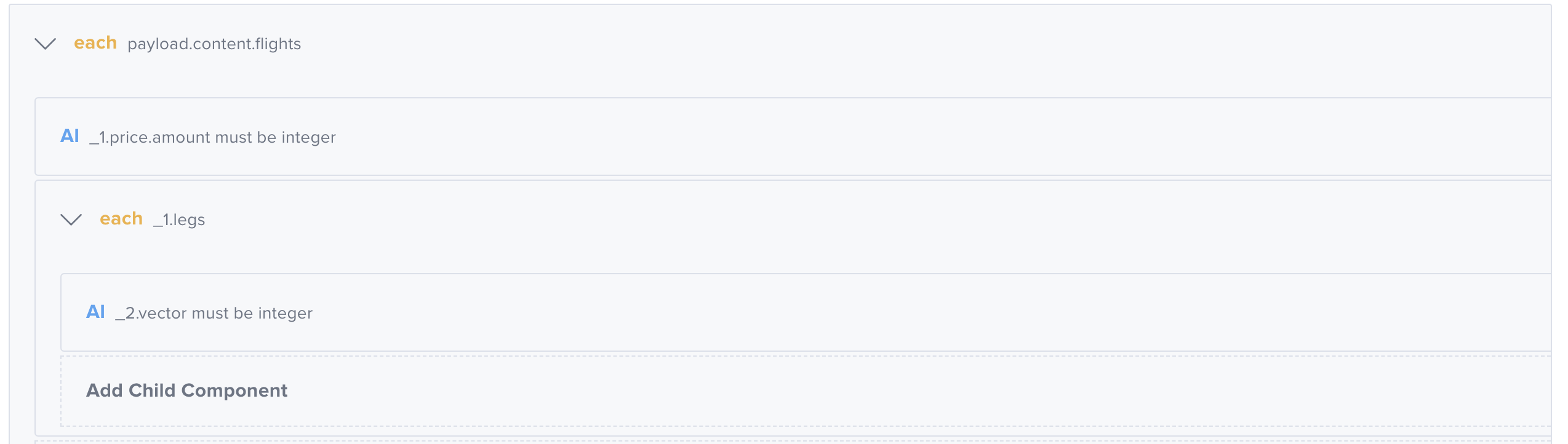
content
item, checks if price.amount
is an integer. Then, for Each legs array, a nested collection in the flights collection, checks if vector
item is an integer value.Nested Collection Example
{
"content": {
"flights": [
{
"price": {
"amount": 100
},
"legs": [
{
"vector": 1
},
{
"vector": 3
}
]
}
]
}
}
Code View Examples
- id: each
children:
- id: assert-is
expression: _1.vector
type: integer
expression: payload.legs
- id: each
children:
- id: assert-is
expression: _1.price.amount
type: integer
- id: each
children:
- id: assert-is
expression: _2.vector
type: integer
expression: _1.legs
expression: payload.content.flights
If
Allows you to run a specific piece of code only if a specific condition is met.
Parameters
Fields | |
| REQUIRED | EXPRESSION | The path of the collection you want to iterate on. |
ExamplesIf
payload.success
is equal to true then the code within the element is executed, otherwise is skipped.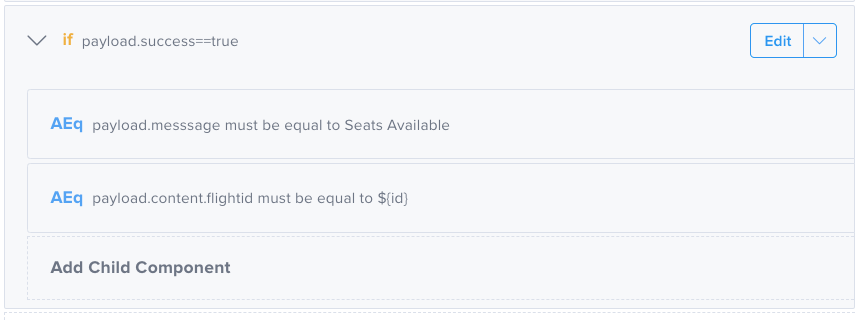
_1.intermediate
exists then the code within the element is executed, otherwise is skipped. This is useful when the element is not always present.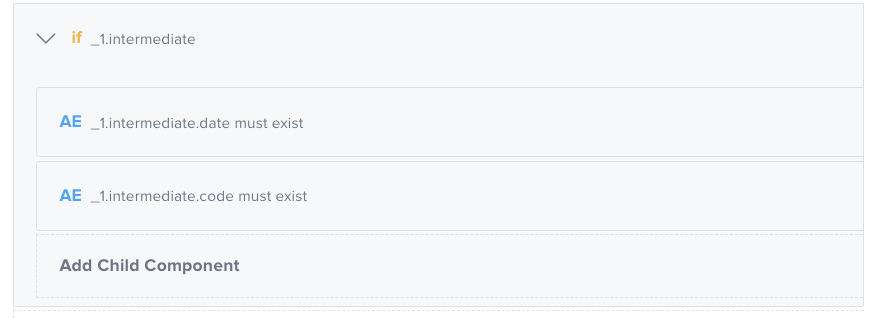
Code View Examples
- id: if
children:
- id: assert-equals
expression: payload.message
value: Seats Available
- id: assert-equals
expression: payload.content.flightid
value: ${id}
type: string
expression: payload.success == true
While
Allows you to run a block of assertions as long as a condition is valid.
Parameters
Fields | |
Expression | | REQUIRED | EXPRESSION | The condition that has to be met for the assertions block to be executed. |
Examples
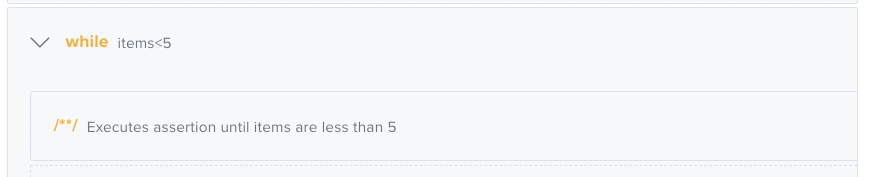
Code View Examples
- id: while
children:
- id: comment
text: Executes assertion until items are less than 5
expression: items<5