Saving a Token in a Key/Value Store
One of the common scenarios you can face when you are working with APIs is authentication. Most of the time you call the endpoint that lets you authenticate and then use the token in the following calls. Sometimes, there may be cases where you can't call the endpoint every time, hence you need to save the token in a variable and use it more times. If you have only one test, you can reuse the same token smoothly. What happens if you need it in more tests inside your project or across the whole organization? The Vault is not the solution because it contains static values and you have to manually update the value every time. Here is when the Key/Value store can help.
What You'll Need
- A Sauce Labs account (Log in or sign up for a free trial license).
- An existing API Testing Project. For details on how to create one, see API Testing Quickstart.
- Familiarity with the API Testing Composer.
Saving a Token in a Key/Value Store
In this example, you learn how to save a token in the Key/Value Store and then use it across the Organization.
First, open the Composer and add the Key/Value Store component.
- Action - for example
Load
- Key - for example
token
- Variable - for example
token_var
It loads the token: the first time you run the test, the value will be empty then, it will be the token retrieved from the following call.
- Action - for example
Click Save Changes.
Add the If component, then Save Changes.
- Expression - for example
!token_var
In this step you check if there is a token in the Key/Value Store: if the token is not available will perform the call to retrieve it, otherwise, the test will proceed using the already available token.
- Expression - for example
Click Add Child Component inside the If and then, add the GET component.
- Url - for example
https://m2-keyvalueexample.load2.apifortress.com/token
- Variable - for example
payload
- Mode - for example
json
This step calls the endpoint that generates the token.
- Url - for example
Click Save Changes.
Add Child Component for the If and then, add the Key/Value Store component.
- Action - for example
Set
- Key - for example
token
- Data - for example
payload.token
In this step you add the value of the token in the Key/Value store.
- Action - for example
Click Save Changes.
Outside the If, add Assert Exists.
- Expression - for example
token_var
token_var
is available for further use inside the test (and inside your Organization).- Expression - for example
Add the POST component and then, Save Changes.
- Url - for example
https://m2-keyvalueexample.load2.apifortress.com/profile
- Variable - for example
profilePayload
- Mode - for example
json
This step calls the endpoint that requires the token for authenticate.
- Url - for example
Add the Request Header to the POST.
- Name - for example
Authorization
- Value - for example
${token_var}
In the request header you pass the token saved in the Key/Value Store.
- Name - for example
The final test looks like:
- id: kv
key: token
action: load
var: token_var
- id: if
children:
- id: get
children: []
url: https://m2-loadtest.load2.apifortress.com/token
var: payload
mode: json
- id: set
var: token_var
mode: string
value: ${payload.token}
- id: kv
key: token
action: set
object: token_var
expression: "!token_var"
- id: assert-exists
expression: token_var
- id: post
children:
- id: header
name: Authorization
value: ${token_var}
url: https://m2-loadtest.load2.apifortress.com/profile
var: profilePayload
mode: json
Using the Key/Value in Other Tests
To use the Key/Value Store in other tests in the same Organization, you have to first load the value from the Key/Value Store and then use it.
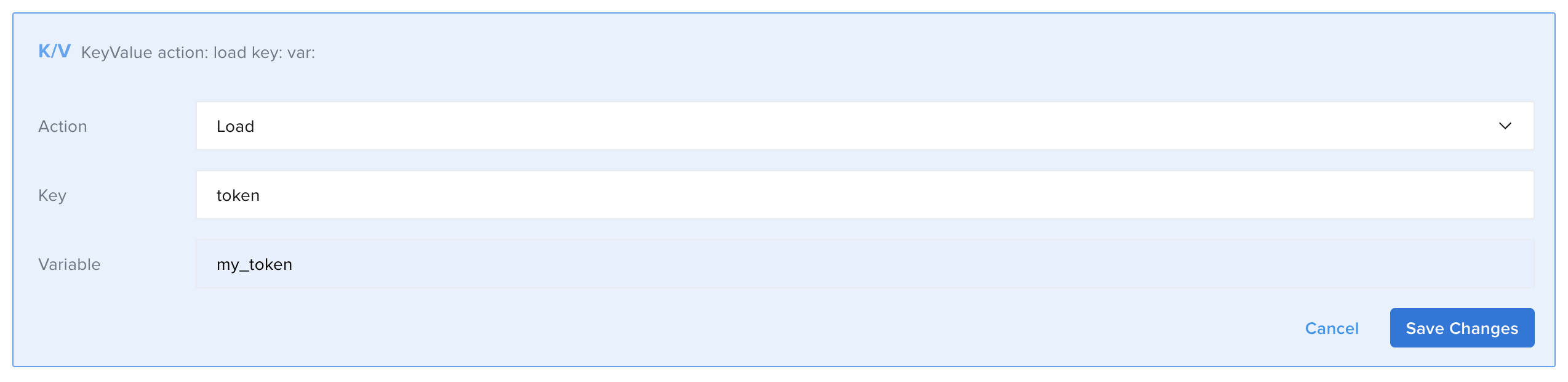
- Action - for example
Load
- Key - for example
token
- Variable - for example
my_token
token
is the Key you assigned in the first step of the previous example.
my_token
is the variable name you will use inside the test for referencing it.
Refreshing the Token
The previous example shows how to save the token in the Key/Value Store but, once created, the token will remain the same. In reality, tokens usually expire after a certain amount of time so you need to generate a new one. In the following example, you will see how to improve the previous example by refreshing the token.
First, add the Key/Value Store component.
- Action - for example
Load
- Key - for example
token
- Variable - for example
token_var
It loads the token: the first time you run the test, the value will be empty then, it will be the token retrieved from the following call.
- Action - for example
Click Save Changes.
Next, add the Key/Value Store component.
- Action - for example
Load
- Key - for example
last_token
- Variable - for example
last_token_var
In this step, you load the date and time the token was last generated.
- Action - for example
Click Save Changes.
Add the If component, then Save Changes.
- Expression - for example
!token_var || ! last_token_var || last_token_var+60000 < D.nowMillis()
In this step you check if there is a token in the Key/Value store, the date is present and one minute has not passed since the last token generation. If any of these conditions are not met, you generate a new one. The example refreshes the token every minute (60000ms), you can choose to refresh it differently by changing the value.
- Expression - for example
Click Add Child Component inside the If and then, add the GET component.
- Url - for example
https://m2-keyvalueexample.load2.apifortress.com/token
- Variable - for example
payload
- Mode - for example
json
This step calls the endpoint that generates the token.
- Url - for example
Click Save Changes.
Add Child Component for the If and then, add the Key/Value Store component.
- Action - for example
Set
- Key - for example
token
- Data - for example
payload.token
In this step you add the value of the token in the Key/Value store.
- Action - for example
Click Save Changes.
Add Child Component inside the If and then, add the Key/Value Store component.
- Action - for example
Set
- Key - for example
last_token
- Data - for example
D.nowMillis()
In this step, you add the date in the Key/Value Store.
- Action - for example
Click Save Changes.
Outside the If, add Assert Exists.
- Expression - for example
token_var
The token will not be generated again for the next minute and the existing one will be used.
- Expression - for example
Add the POST component and then, Save Changes.
- Url - for example
https://m2-keyvalueexample.load2.apifortress.com/profile
- Variable - for example
profilePayload
- Mode - for example
json
- Url - for example
This step calls the endpoint that requires the token for authenticate.
Add the Request Header to the POST.
- Name - for example
Authorization
- Value - for example
${token_var}
In the request header you pass the token saved in the Key/Value Store.
- Name - for example
The test looks like:
- id: kv
key: token
action: load
var: token_var
- id: kv
key: last_token
action: load
var: last_token_var
- id: if
children:
- id: get
children: []
url: https://m2-keyvalueexample.load2.apifortress.com/token
var: payload
mode: json
- id: kv
key: token
action: set
object: payload.token
- id: kv
key: last_token
action: set
object: D.nowMillis()
expression: "!token_var || ! last_token_var || last_token_var+60000 < D.nowMillis()"
- id: assert-exists
expression: token_var
- id: post
children:
- id: header
name: Authorization
value: ${token_var}
url: https://m2-keyvalueexample.load2.apifortress.com/profile
var: profilePayload
mode: json
By running the test two times in a row (in less than one minute) you can notice that the first time, the test will perform the call that generates the token and the second time it will execute only the call that uses the token.